Module Definition and Initialization
Now that we have defined our module, let's create a couple of files that are needed by activeCollab to recognize and load the module. First set the module image. For this we need to create a folder /path/to/activecollab/custom/modules/my_reports/assets/default/images and place our image in it. It should be a 32x32 pixel PNG icon called module.png.
Initialization File (init.php) #
Next, write the module initialization file by creating an empty init.php file inside the /path/to/activecollab/custom/modules/my_reports folder.
Use the following code:
1 2 3 4 5 6 7 8
<?php /** * My Reports module initialization file */ const MY_REPORTS_MODULE = 'my_reports'; const MY_REPORTS_MODULE_PATH = __DIR__;
Signature File (signature.php) #
Since version activeCollab 3.3, every module requres a valid signature file. To define one, please create a signature.php file in your /modules/the-module-you-made folder.
This file needs to be in the following format:
1 2 3 4 5 6
<?php /** * Return module version signature */ return 'qw13XUlqFb2b^0R8)u<DH3nx*L6DY3>C06K7]D3M';
The purpose of a signature file is to let activeCollab know that a module is compatible with a particular version of the system. If the signature file is missing, or it returns an invalid value, the system will simply skip the module and will not load it at all.
Each major branch of activeCollab (marked by the first two numbers in version number, eg. 1.0, 3.2, 5.6 etc) will have a different signature value. When a new branch is released, you will need to review your module and update the module signature file. By using this method, we wish to help you keep your modules up to date, reviewed and compatible with the latest activeCollab version branch.
Currently the known signature values are:
- activeCollab 3.3 - 'TcFnJCm<jPNihLiGGzizrMUzh6:%6sEx79U2<#Vz' (without quotes)
- activeCollab 4.0 - 'qw13XUlqFb2b^0R8)u<DH3nx*L6DY3>C06K7]D3M' (without quotes)
- activeCollab 4.1 - '2D4xeWJpEI7p6uqf6nTNLHYweculoPe7133n8fH6' (without quotes)
- activeCollab 4.2 - '2D4xeWJpEI7p6uqf6nTNLHYweculoPe7133n8fH6' (without quotes)
Module Definition Class #
The next step is to create a module definiton class. By convention, the module definition class is named the same way as the module folder, but in camel notation plus the word "Module". Here are some examples:
- reports will be ReportsModule
- sales_force_integration will be SalesForceIntegrationModule
- ldap_provider will be LdapProviderModule
Save the module definition class to a file that has the same name as the definition class and add ".class.php" extension to it. In our case, we will add MyReportsModule.class.php to the folder /path/to/activecollab/custom/modules/my_reports.
The code in MyReportsModule.class.php will look something like this:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54
<?php /** * My Reports module definition class */ class MyReportsModule extends AngieModule{ /** * Short module name (should be the same as module folder name) * * @var string */ protected $name = 'my_reports'; /** * Module version * * @var string */ protected $version = '1.0'; /** * Return module name (displayed in activeCollab administration panel) * * @return string */ function getDisplayName() { return lang('My Reports'); } /** * Return module description (displayed in activeCollab administration panel) * * @return string */ function getDescription() { return lang('An example module'); } /** * List events that this module listens to and define event handlers */ function defineHandlers() { // Place where you can define your event handlers } /** * List routes defined and used by this module */ function defineRoutes() { // Place where you can define your routes } }
Install the Module #
Now that we have our module icon, initialization and signature files, and the module definition class, our new module will be available for installation. Use the Refresh in the Administration to be able to see the module in the Modules list:
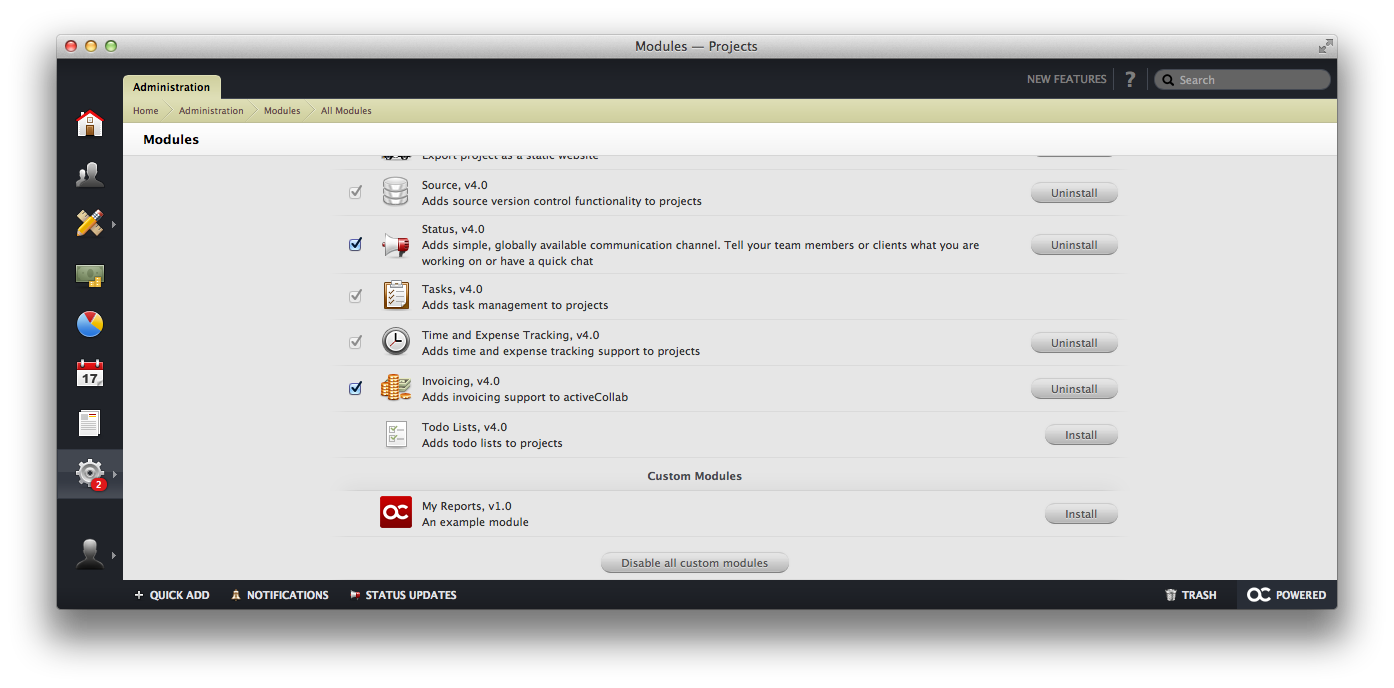
After completing all the steps described in this article, this is how the /modules folder structure should look like:
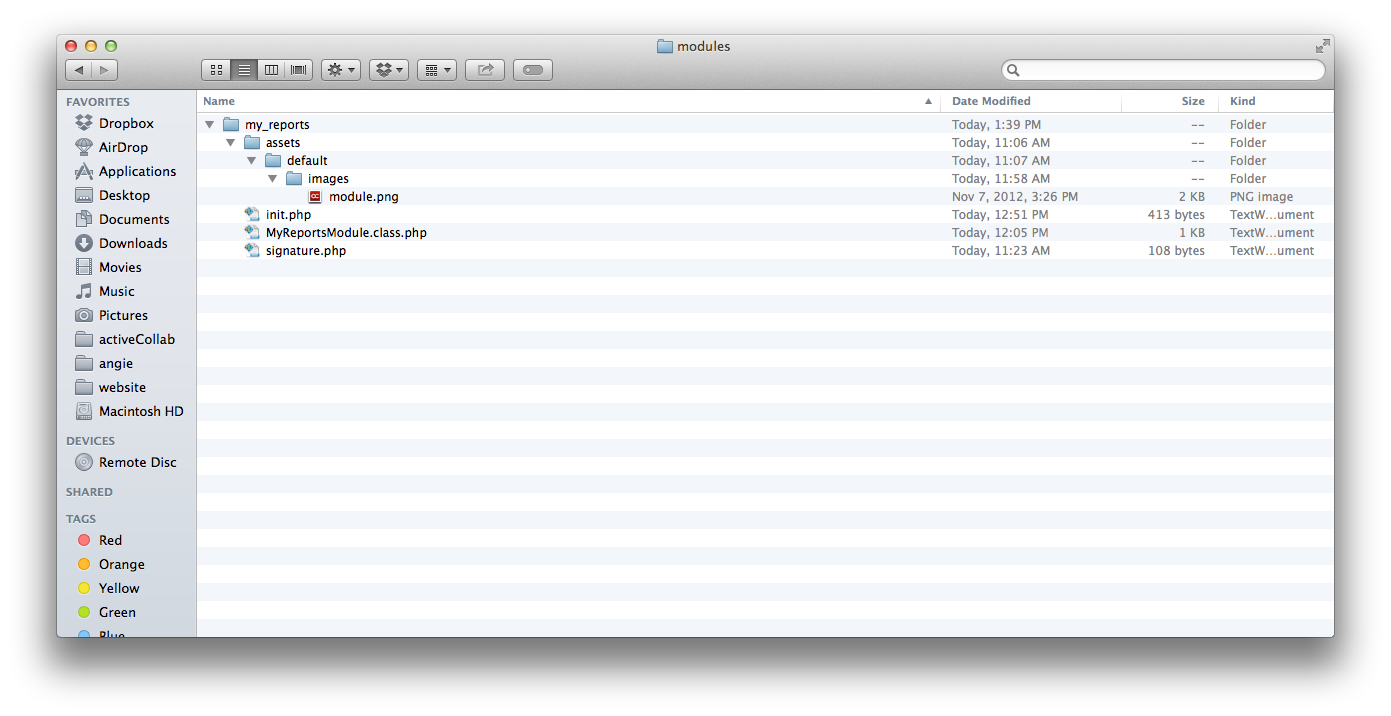